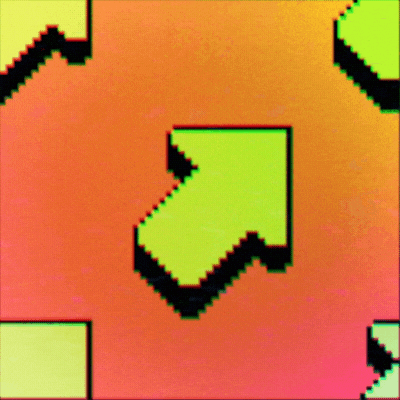
With vanilla javascript, we would typically declare functions with the following syntax:
const myFunction = function(params1, params2, etc..){
//do something here
}
Pretty straight forward, wouldn’t you say?
Then comes along the arrow function, or whatever you want to call it. Some have called it rocket arrow, while one site I came across called it the fat arrow.
The arrow function syntax was introduced in ES6 in hopes of making writing syntax for functions simpler and cleaner. A version of the function above using arrow functions would look something like this:
const myFunction = (params1, params2, etc...) => //do that thing you do
For simple functions, it may not make a difference, but as the functions grow larger, it might!
Some other details about arrow function syntax
Arrow functions without parameters can be written like so:
() => { /* do something here */ }
When there’s only one parameter to pass, opening parenthesis are optional:
paramaters => { /* JUST DO IT */ }
(paramaters) => { /* SPONSORED BY NIKE */ } //effectively the same thing as above
If your function is returning an object literal, be sure to wrap it in parenthesis:
/* No bueno. It'll think it's a regular function body */
(name, description) => {name: name, description: description};
/* That's better */
(name, description) => ({name: name, description: description});
This…again
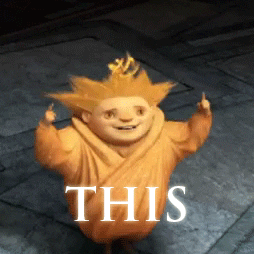
One other thing that arrow functions have is it’s own ‘this’. Instead, by using an arrow function, the ‘this’ is bound to wherever the function is defined. Using React as an example, let’s say we have a button within a component that has a function that changes the background color, but the function has regular syntax and not arrow syntax.
/* Within the render method */
return (
<button onClick={this.handleClick} style={this.state}>
Set background to red
</button>
)
/* function written normally * /
handleClick() {
this.setState({ backgroundColor: 'red' })
}
Written this way, the ‘this’ within handleClick function would be null. Had handleClick() been written as an arrow function, like so:
handleClick = () => {
this.setState({ backgroundColor: 'red' })
}
then ‘this’ would be hard-wired refer to the location at which the function was defined, in this case, the class component that the function is within.
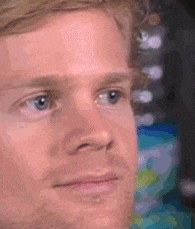
Confusing, I know. However, using arrow functions does save some guesswork when it comes to figuring out ‘this’ bugs and following the trails of callback methods.
Thanks for reading my article. Hope you like it!